How to Convert PNGs to JPGs in 7 Lines of Python
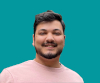
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Python is a powerful programming language that can be used to perform a wide range of tasks. One of those tasks is image manipulation, which can be done using the Pillow library. Although it is possible to convert a PNG file to a JPEG file manually, it can be tedious and time-consuming. Fortunately, with a few lines of Python code, you can convert all the PNGs in a directory to JPEGs while retaining the quality of the photo.
1. Install Pillow
The first step is to install Pillow, which is a fork of the Python Imaging Library (PIL). Pillow is an easy-to-use library that provides support for image manipulation and processing. To install Pillow, open a terminal window and run the following command:
pip install Pillow
Once the installation is complete, you are ready to proceed with the next step.
2. Import Required Modules
Once Pillow is installed, you need to import the necessary modules into your program. To do this, add the following lines of code at the beginning of your program:
from PIL import Image
import os
The first line imports the Image module from Pillow, while the second line imports the os module which provides access to operating system functionality.
3. Get the List of Files in the Directory
Next, you need to get a list of all the PNG files in the directory that you want to convert into JPEGs. To do this, you can use the os module’s listdir() function:
files = os.listdir('path/to/directory') # list of files in directory
This will return a list of filenames as strings. You can then loop through this list and check if each one is a PNG file:
for file in files:
if file.endswith('.png'): # check if file is a png
# code for converting png to jpg goes here
4. Open and Save Files as JPEGs
Now that you have identified all the PNG files in the directory, you can open each one using the Image module’s open() function:
im = Image.open(file).convert("RGB") # open file as an image object
Once you have opened the image object, you can then save it as a JPEG using the save() function:
im.save('path/to/directory/jpgs/' + file[:-4] + '.jpg') # save image as jpg
When saving the image as a JPEG, you can also specify optional parameters such as quality and optimization:
im.save('path/to/directory' + file[:-4] + '.jpg', quality=95, optimize=True) # save image as jpg with options
5. Put it All Together
When we put all the previous steps together it should look like this:
from PIL import Image
import os
files = os.listdir('path/to/directory') # list of files in directory
for file in files:
if file.endswith('.png'): # check if file is png
im = Image.open(file).convert("RGB") # open file as an image object
im.save('path/to/directory' + file[:-4] + '.jpg', quality=95, optimize=True) # save image as jpg with options
And that’s it! With just a few lines of Python code, you can convert all PNGs in a directory to JPEGs while retaining quality.
Find the code in my GitHub here.
Watch the video tutorial here:
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!