How to Create a Simple Animation with the Framer Motion Library in React.js
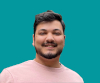
Jacob Naryan - Full-Stack Developer
Posted: Wed Nov 22 2023
Last updated: Wed Nov 22 2023
Creating animations in React.js can be a daunting task, especially for beginners. That’s where the Frame Motion library comes in. This powerful yet easy-to-use tool makes it simple to add intricate animations to your React.js projects. In this tutorial, we’ll walk you through the steps to create a simple animation using Framer Motion.
1. Setting Up the Project
First things first, you need a new React project. If you have Node.js and npm installed, you can create a new project using the Create-React-App tool:
npx create-react-app frame-motion-demo
After creating your new project, navigate into its directory:
cd frame-motion-demo
2. Installing Framer Motion
Next, install Framer Motion. You can do this using npm:
npm install framer-motion
3. Importing Framer Motion
In your React.js component file, import the motion
component from the framer-motion
package:
import { motion } from 'framer-motion';
The motion
component is a magical tool that will allow us to animate any HTML or SVG element.
4. Creating Your Animation
Now it’s time to add an animation! Let’s create a simple motion.div element that scales from 0 to 1 and rotates 180 degrees:
<motion.div
initial={{ scale: 0 }}
animate={{ rotate: 180, scale: 1 }}
transition={{
type: "spring",
stiffness: 260,
damping: 20
}}
/>
- The
initial
prop sets the initial state of the animation. - The
animate
prop sets the final state of the animation. - The
transition
prop sets the type of animation and its properties, such as stiffness and damping.
5. Running Your Animation
To see your animation in action, start your React development server:
npm start
With that, you should see your animation in action! The div will start from a scale of 0, rotate by 180 degrees, and then scale up to 1.
Conclusion
Framer Motion is a powerful tool for creating animations in React.js. With just a few lines of code, you can add impressive animations to your app. This tutorial only scratches the surface of what you can do with Framer Motion, so be sure to check out the official documentation for more information.
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
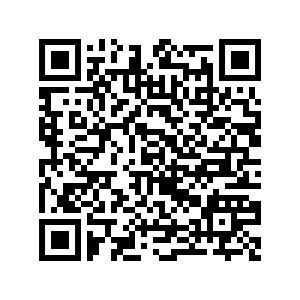