How to Find The Most Recent File in a Directory in Python
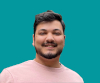
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
There are many reasons why you might need the most recent file in a directory. You may need to run some code every time a file is uploaded to a certain directory or some other process. This snippet can be used in a variety of programs and is a good resource to bookmark for later.
1. Import os Library
The first step is to import the os library, which will provide access to the directory path and the files within it.
import os
2. Set the Directory Path
Next, set the directory path, which is the location of the directory you want to search.
directory_path = '/path/to/directory/'
3. Initialize Variables
Before you start looping through the files, create and initialize two variables to hold the name of the most recently modified file and its modification time.
most_recent_file = None
most_recent_time = 0
4. Iterate Over Files Using os.scandir()
Now you can loop through the files in the directory using os.scandir(), which returns an iterator object containing all of the files in the directory.
# iterate over the files in the directory using os.scandir
for entry in os.scandir(directory_path):
if entry.is_file():
# get the modification time of the file using entry.stat().st_mtime_ns
mod_time = entry.stat().st_mtime_ns
if mod_time > most_recent_time:
# update the most recent file and its modification time
most_recent_file = entry.name
most_recent_time = mod_time
5. Do Something with The File
Finally, do something with the most recently modified file, such as open it, write to it, or copy it to another location.
# open the file for reading
with open(most_recent_file, 'r') as f:
# read file contents into a variable
contents = f.read()
# do something with contents of file
# write contents back to file
f.write(contents)
# close file when done reading/writing
f.close()
Watch the video tutorial here:
Thank you for reading. If you liked this blog, check out my personal blog for more content like this.