How to Implement a Simple Payment Field with Square Payments and React
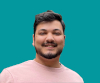
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
The ability to quickly and easily process payments is an important part of any website or mobile application. Square Payments and React make it possible for developers to integrate a payment field into their projects with ease. In this blog post, we’ll walk through step-by-step how to implement a simple payment field with Square Payments and React.
1. Get a Square Account
The first step is to create a Square account. This can be done by visiting squareup.com and creating an account. Once you have an account, you’ll need to create an application within the Developer Dashboard, which can be accessed from your Square Account’s dashboard.
2. Create an Application within the Developer Dashboard
Once you’ve created an account and logged in, you’ll need to create an application within the Developer Dashboard. This can be done by clicking the “Create New Application” button at the top of the page. You’ll then need to fill out the form with your application’s name, description, URL, and other relevant details before submitting it for review.
3. Add the PaymentForm Component to Your React Project
Once your application has been approved, you’ll need to add the PaymentForm component JavaScript file to your React project. This file can be found in the Square PaymentForm SDK repository on GitHub. To add it to your project, simply copy and paste the code into your project’s source code. Or, include this script somewhere in your project:
<script src='https://js.squareup.com/v2/paymentform'></script>
4. Render the PaymentForm Component in Your App Component
Now that you have the PaymentForm component in your project, you’ll need to render it in your App component. To do this, you’ll need to import the PaymentForm component into your App component file and then use the <PaymentForm />
tag in your App component's render method.
Below is an example of how this should look:
import React from 'react';
import PaymentForm from './PaymentForm'; // Import PaymentForm component
class App extends React.Component {
render() {
return (
<div className="App">
<PaymentForm /> // Render PaymentForm Component
</div>
);
}
}
5. Test Your Payment Form
Once you’ve added your PaymentForm component and rendered it in your App component, you’re ready to test it out! To do this, simply run your app using npm start
or whatever command you use to run your React app and then fill out the payment form with some test data (credit card numbers, etc.). If all goes well, you should see a confirmation message once you've submitted the form!
And that’s all there is to it! With just a few steps, you can quickly and easily integrate a payment field with Square Payments and React into your project. Thank you for reading. If you liked this blog, consider following my Medium account for daily blogs about software engineering and technology topics!
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
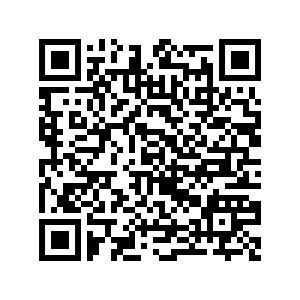