How To Make Get and Post Requests with JavaScript
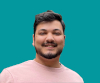
Jacob Naryan - Full-Stack Developer
Posted: Sat Jul 22 2023
Last updated: Wed Nov 22 2023
Fetch is a modern web API that makes it easy to make HTTP requests from within JavaScript. It provides an interface for making both GET and POST requests, allowing for more complex network requests than other built-in methods. This tutorial will teach you how to make both types of requests with Fetch.
1. Setting Up Fetch
Before you can use Fetch, you will need to check if the browser supports it. Most modern browsers do, but if you need to support older browsers, you may need to use a polyfill.
Once you have determined that your browser supports Fetch, you can start writing code. The syntax for making a request is simple:
fetch(url, {options}).then(function(response) {
// handle response here
});
The first parameter is the URL of the request, and the second parameter is an optional object containing options for the request. We will discuss these options in more detail later on.
2. Making a GET Request
A GET request is used to retrieve data from a server. To make a GET request with Fetch, all you need to do is pass in the URL as the first parameter:
fetch('https://www.example.com/data').then(function(response) {
// handle response here
});
This will make an asynchronous request to the specified URL and return a response object. The response object has several properties that can be used to determine the status of the request (e.g., status code, headers), as well as methods for reading the data sent back by the server (e.g., text(), json()).
3. Making a POST Request
A POST request is used to send data to a server. To make a POST request with Fetch, you need to pass in an object as the second parameter with the method property set to POST and the body property set to the data you want to send:
fetch('https://www.example.com/data', {
method: 'POST',
body: JSON.stringify({name: 'John Doe'}) // stringify data before sending
}).then(function(response) {
// handle response here
});
This will make an asynchronous POST request with the specified data, and return a response object that can be used to determine the status of the request and read any data sent back by the server.
4. Additional Request Options
There are several other options that can be passed into Fetch when making a request, such as headers, credentials, cache, and mode. These options can be used to customize the details of your requests, such as setting custom headers or specifying credentials (e.g., cookies). You can find more information about these options on MDN.
5. Conclusion
In this tutorial, we have learned how to use Fetch to make both GET and POST requests in JavaScript. We have also discussed some of the additional options that can be used to customize your requests. Using Fetch makes it easy to make complex network requests from within JavaScript, allowing you to quickly build powerful web applications.
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.