How to Set and Retrieve Session Variables in .NET
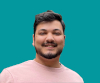
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
Session variables play a crucial role in preserving user data across multiple requests on the server. They enable the server to track client interaction and state. This blog post will guide you on how to set and retrieve session variables in a C# API controller using Dependency Injection and the IHttpContextAccessor class.
1. Setting up the Startup.cs
Before we delve into setting and retrieving session variables, let’s configure our Startup.cs
file to support session state.
public void ConfigureServices(IServiceCollection services)
{
services.AddSession();
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor(); // Adds IHttpContextAccessor to DI
}
In Configure
method:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
...
app.UseSession(); // Enables session for the application
...
}
In the ConfigureServices
method, we add services for Session. We also inject an instance of IHttpContextAccessor into our application.
In the Configure
method, we use the UseSession()
method to enable sessions for the application.
2. Creating an API Controller
After setting up Startup.cs
, let's create an API controller where we will set and retrieve session variables using Dependency Injection and the IHttpContextAccessor class.
[ApiController]
public class SessionController : ControllerBase
{
private readonly IHttpContextAccessor _httpContextAccessor;
public SessionController(IHttpContextAccessor httpContextAccessor)
{
_httpContextAccessor = httpContextAccessor;
}
}
We have injected IHttpContextAccessor
into our controller using the constructor.
3. Setting Session Variables
Now, let’s create a method inside of our SessionController
that will set a session variable:
[HttpPost]
public IActionResult SetSessionValue([FromBody] string value)
{
_httpContextAccessor.HttpContext.Session.SetString("MySessionKey", value);
return Ok();
}
Here, we’ve created a POST endpoint SetSessionValue
. It accepts a value from the request body and sets it into session using key "MySessionKey".
4. Retrieving Session Variables
To retrieve our session variable, we’ll create another method:
[HttpGet]
public IActionResult GetSessionValue()
{
var value = _httpContextAccessor.HttpContext.Session.GetString("MySessionKey");
return Ok(value);
}
This GET endpoint GetSessionValue
retrieves the value from the session using the key "MySessionKey".
And there you have it! You can now set and retrieve session variables within your C# API controllers using Dependency Injection and IHttpContextAccessor. This simple yet powerful functionality can greatly assist in creating stateful API interactions.
Thank you for reading. If you liked this blog check out more of my tutorials about software engineering on my personal site here.