How to Setup Angular Router in Your Angular Application
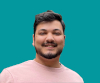
Jacob Naryan - Full-Stack Developer
Posted: Fri Mar 31 2023
Last updated: Wed Nov 22 2023
Angular Router is one of the best solutions for setting up routing in your single-page application. In this tutorial, I will show you how to implement Angular Router into your Angular application.
1. Install the Angular Router
In order to set up routes in Angular, you need to install the Angular Router. You can do this by running the following command:
npm install @angular/router --save
2. Import the Router Module
Once the router has been installed, you need to import it in your root module (typically app.module.ts). To do this, add the following import statement:
import { RouterModule } from '@angular/router';
import { Routes } from '@angular/router';
Then, add RouterModule to the imports array of your module:
@NgModule({
imports: [
RouterModule
]
})
3. Configure Routes
The next step is to configure the routes for your application. To do this, define an array of routes in your module and then pass it to the forRoot() method of RouterModule. For example:
const routes: Routes = [
{ path: '', component: HomeComponent }, // Home page route
{ path: 'about', component: AboutComponent } // About page route
];
@NgModule({
imports: [
RouterModule.forRoot(routes) // Add routes to the app
]
})
4. Add a Router Outlet to Your App Component Template
Finally, you need to add a <router-outlet></router-outlet> element to your application's root component template (typically app.component.html). This is where the components for the various routes will be rendered. For example:
<div class="container">
<router-outlet></router-outlet> <!-- Add router outlet -->
</div>
And that’s it! You have now successfully configured routes in Angular with Angular Router.
Thank you for reading. Check out more tutorials about software engineering here.