How to Tell if an HTML Element is in The Viewport
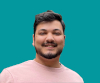
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
When adding animations to a website with CSS, HTML, and JavaScript, you may need to know if an element is in view to trigger the animation. In order to determine if an HTML element is visible on the page, you can use a function to check if the element is within the current viewport of the browser window. This tutorial will walk you through how to create a custom JavaScript function that you can use to check if an element is visible or not.
2. Create the Function
To create the function, you will need to start by declaring it. In this example, we will be declaring the function as isInViewport
. The code for this should look like this:
function isInViewport(el) {
// ...
}
3. Get the Bounding Client Rectangle
In order to determine if an element is in the viewport, we need to get its bounding rectangle. You can do this using the getBoundingClientRect()
method on the HTML element. The code should look something like this:
function isInViewport(el) {
const rect = el.getBoundingClientRect();
}
4. Check the Element’s Position
Now that we have the bounding rectangle of the element, we can use it to check its position relative to the viewport. To do this, we need to compare the top, left, bottom, and right positions of the rectangle to those of the viewport. The code should look like this:
function isInViewport(el) {
const rect = el.getBoundingClientRect();
var isinview = (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
return isinview;
}
The variable isinview
will be set to true
if all of these conditions are met - meaning that the element is in view. If any of these conditions are not met, then isinview
will be set to false
, meaning that the element is not in view.
5. Return the Result
Once all of these conditions have been checked, we can return our result by returning the value of isinview
. The code should look like this:
function isInViewport(el) {
const rect = el.getBoundingClientRect();
var isinview = (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
// returns true or false based on whether or not the element is in viewport
return isinview;
}
And that’s it! Now you have a custom JavaScript function that you can use to check if an HTML element is visible or not!
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!