How to Write a Simple API with Entity Framework
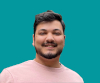
Jacob Naryan - Full-Stack Developer
Posted: Fri Mar 31 2023
Last updated: Wed Nov 22 2023
Entity framework is an awesome framework to interact with your database if you have a simple application with a small to medium amount of data. With Entity Framework, you can make an API that allows you to add, update, read, and delete data very easily. In this tutorial, we will show you how to do just that.
1. Creating a Record
Using Entity Framework, we can easily create a record in a simple SQL table with 3 fields: id, name, and email. First, we need to create an object to represent the data we are trying to store.
public class MyRecord
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
Next, we need to create our database context, which serves as the bridge between our code and the database.
public class MyDatabaseContext : DbContext
{
public DbSet<MyRecord> Records { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer("Server=localhost;Database=MyDatabase;Trusted_Connection=True;");
}
}
Make sure to replace the details above with your database details.
Finally, we can use the database context to add a new record to the table.
using (var dbContext = new MyDatabaseContext())
{
var myRecord = new MyRecord
{
Name = "John Smith",
Email = "john.smith@example.com"
};
dbContext.Records.Add(myRecord);
dbContext.SaveChanges();
// myRecord.Id has now been populated with the ID of the record in the database
}
2. Reading a Record
We can also use Entity Framework to read records from our simple SQL table. To do this, we will use the Find method on our database context. This method takes the ID of the record that we want to find as an argument and returns an object of type MyRecord.
using (var dbContext = new MyDatabaseContext())
{
// Finds the record with ID 1
var myRecord = dbContext.Records.Where(x => x.Id == 1).FirstOrDefault();
if (myRecord != null) // Check if a record was found
{
Console.WriteLine($"Name: {myRecord.Name}, Email: {myRecord.Email}");
}
// Outputs: Name: John Smith, Email: john.smith@example.com
}
3. Updating a Record
We can also use Entity Framework to update an existing record in our database table. To do this, we first need to retrieve the record that we want to update using the `FirstOrDefault` method. Then, we can modify the properties of the object and call `SaveChanges` on our database context to persist the changes to the database.
using (var dbContext = new MyDatabaseContext()) {
// Finds the record with ID 1
var myRecord = dbContext.Records.Where(x => x.Id == 1)FirstOrDefault();
// Check if a record was found
if (myRecord != null) {
myRecord.Name = "Jane Smith";
myRecord.Email = "jane.smith@example.com";
dbContext.SaveChanges();
}
}
4. Deleting a Record
Finally, we can use Entity Framework to delete an existing record from our database table by using the `Remove` method on our database context. This takes an object of type `MyRecord`, so we must first retrieve it using the `FirstOrDefault` method as before, and then pass it into `Remove`. Finally, calling `SaveChanges` will persist the deletion to the database table.
using (var dbContext = new MyDatabaseContext())
{
// Finds the record with ID 1
var myRecord = dbContext.Records.Where(x => x.Id == 1).FirstOrDefault();
if (myRecord != null)
{
// Removes the records and saves changes
dbContext.Remove(myRecord);
dbContext.SaveChanges();
}
}
Thank you for reading. If you liked this blog check out more tutorials about software engineering here.
Thanks for reading, please consider tipping some BTC if you liked the blog!
Click or scan the QR code below.
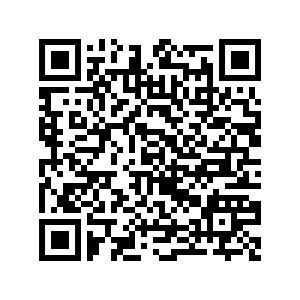