Make an Audio Voiceover of Any Text File With 7 Lines of Python
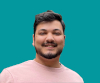
Jacob Naryan - Full-Stack Developer
Posted: Sat Aug 05 2023
Last updated: Wed Nov 22 2023
If you’ve ever wanted to add a voiceover to your videos, you might have found that it can be time-consuming and costly to hire a professional voice actor. Luckily, there’s a simple and affordable way to create a voiceover for your videos: using Python and the gTTS
library.
gTTS
(Google Text-to-Speech) is a Python library that allows you to convert text to speech using Google's Text-to-Speech API. In this tutorial, we'll walk through how to use gTTS
to easily create an audio voiceover for any text file.
Getting Started
Before we begin, you’ll need to have Python installed on your computer. You can download and install the latest version of Python from the official Python website (https://www.python.org/downloads/).
You’ll also need to install the gTTS
library. You can install it using pip, which is a package manager for Python. Open a terminal or command prompt and type the following command:
pip install gTTS
This will install the gTTS
library and any necessary dependencies.
Writing Your Text
The first step is to write the text that you want to convert to an audio voiceover. You can use any text editor, such as Notepad, Sublime Text, or Atom, to write your text. Save your text as a plain text file with the file extension .txt
.
For this tutorial, we’ll create a file named example.txt
with the following text.
Python is a high-level programming language that is easy to learn and widely used. It is used for web development, data analysis, artificial intelligence, and more
Converting Text to Audio
Now that we have our text file, we can use gTTS
to convert the text to an audio voiceover. Open a new Python file and import the gTTS
library:
from gtts import gTTS
Next, we’ll read the contents of the text file and store them in a variable:
filename = "example.txt"
with open(filename, "r") as file:
# Read the contents of the file into a string
txt = file.read()
The open
function is used to open the text file and the with
statement ensures that the file is properly closed after we're done with it. The read
function is used to read the contents of the file and store them in the txt
variable.
Now, we’ll specify the language that we want to use for the voiceover. In this example, we’ll use English:
language = 'en'
Finally, we’ll use the gTTS
function to convert the text to an audio voiceover and save it as an MP3 file:
myobj = gTTS(text=txt, lang=language, slow=False)
myobj.save("example.mp3")
The text
parameter is used to specify the text that we want to convert, the lang
parameter is used to specify the language that we want to use, and the slow
parameter is used to specify whether the audio should be generated slowly or quickly. In this case, we're using the default value of slow=False
, which generates the audio quickly.
Conclusion
That’s it! With just a few lines of Python, we’ve converted a text file to an audio voiceover that can be used in our videos.
Thank you for reading. If you liked this blog, check out my personal blog for more content about software engineering and technology topics!
If you want to watch a video tutorial on how I did this from start to finish, I made a YouTube video here: